A complete example, this can be in a __tests__/myFunctionTest.ts file: Note this is very very close to the mock you need for Express - the firebase functions request / response are built on those Typescript interfaces so the strategy should apply. Not the answer you're looking for? (filename, data), // TypeScript is currently the only supported language, // Specific interfaces to write to output, // One of object|json|string. Rather than handcrafting an object that implements all the methods I'm looking for a library to do that for me. Mastery though, is not the end goal. Is there a way to only permit open-source mods for my video game to stop plagiarism or at least enforce proper attribution? Use a type assertion first to unknown then to the interface you want in order to make the compiler accept it. // have been called by looking commands invoked on the mocks. To subscribe to this RSS feed, copy and paste this URL into your RSS reader. Another note Khalil. Browse other questions tagged, Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide. It also means our tests and test doubles will be brittle since adding new methods to an interface requires changing the test doubles. 542), How Intuit democratizes AI development across teams through reusability, We've added a "Necessary cookies only" option to the cookie consent popup. How to convert a string to number in TypeScript? Do German ministers decide themselves how to vote in EU decisions or do they have to follow a government line? There was a problem preparing your codespace, please try again. Having to provide an implementation everytime you create a test double leads to brittle tests. Use Git or checkout with SVN using the web URL. If you do not want to specify types at all, TypeScripts contextual typing can infer the argument types since the function value is assigned directly to a variable of type SearchFunc. One final way to get around these checks, which might be a bit surprising, is to assign the object to another variable: In this post, I'll explain how many of us are not actually mocking properly using Jest, what some of the implications of that are, and how to fix it. Type '(src: string, sub: string) => string' is not assignable to type 'SearchFunc'. The whole point is to have a convenient way to generate a mock given an interface, so that developers don't have to manually create mock classes just to, say, stub out a single function out of a dozen methods every time you need to run a test. If you have used before a library like Moq then the syntax should look familiar, otherwise the Refresh the page, When creating mock instances out of global objects (such as window.localStorage), you should provide the name of the global object ("localStorage" in this case) as the second parameter. Here, also, the return type of our function expression is implied by the values it returns (here false and true). You'll build a solid foundation based on the most important parts of topics like: Domain-Driven Design, Test-Driven Development, BDD, Object Design, Functional Programming, Design Principles, Design Patterns, Architectural Styles, Deployment Pipelines and more. Argument of type '{ colour: string; width: number; }' is not assignable to parameter of type 'SquareConfig'. Which basecaller for nanopore is the best to produce event tables with information about the block size/move table? Last week I was creating a NodeJS + ExpressJS app in TypeScript and I was wondering how to apply the Onion Architecture successfully. By clicking Accept all cookies, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy. rev2023.3.1.43269. Any amount is appreciated! rev2023.3.1.43269. How to convert a string to number in TypeScript? You can do that of course, check the parameters, but I think rather than mocking, you would be better off using integration testing to verify the parts of your application that involve side effects such as updating the database and sending an email. So mathlib.multiplier just needs to be assigned to an object that conforms to IMultiplier. Find centralized, trusted content and collaborate around the technologies you use most. An interface can extend multiple interfaces, creating a combination of all of the interfaces. Is it possible to mock a typescript interface with jest? Use a type assertion first to unknown then to the interface you want in order to make the compile Do German ministers decide themselves how to vote in EU decisions or do they have to follow a government line? What I needed was the ability to merely specify the interface of a mock object and let the testing framework create the mock for me. Variables use const whereas properties use readonly. Share it on Social Media. You can still override it with a type assertion, though: The easiest way to remember whether to use readonly or const is to ask whether youre using it on a variable or a property. You will have a problem if you want to actually call a method or get the value of a property in your mock object. Otherwise the TypeScript compiler will omit the uninitialized property from the emitted JavaScript and hence TypeMoq will throw a MockException with an 'invalid setup expression' message. It's very likely to change over the next little while. Note: During the creation of the static mock, the target object is being instantiated as a regular JavaScript object by executing the target constructor with any provided constructor args, Note: To create the static mock, the provided target object is replaced by a deep clone which is accesible through the .target property of the resulting mock object. In our example this would cause the typescript compiler to emit an error on line 9 saying: How to convert a string to number in TypeScript? How did Dominion legally obtain text messages from Fox News hosts? Connect and share knowledge within a single location that is structured and easy to search. Does Cast a Spell make you a spellcaster? E.g.. Type 'Clock' provides no match for the signature 'new (hour: number, minute: number): any'. in jest we use 'spyOn' for this so there's already a clash, b) mock - is when we provide alternative implementations (with empty function as a default) for the whole module. Difference between the static and instance sides of classes. Add a new jest.config.js file to the root of your project: 1 To subscribe to this RSS feed, copy and paste this URL into your RSS reader. WebThe mock just needs to have the same shape as the interface. Here, we show how you can create a variable of a function type and assign it a function value of the same type. See how TypeScript improves day to day working with JavaScript with minimal additional syntax. Allow to verify a mock method call never occurred in Strict mode. rev2023.3.1.43269. Interfaces are a concept in TypeScript that only exist during compile time. Object literals get special treatment and undergo excess property checking when assigning them to other variables, or passing them as arguments. Simple mocking library for JavaScript targeting TypeScript development. Not the answer you're looking for? Compared to static global mocks, dynamic global mocks suffer from the same limitations as regular dynamic mocks. It will however, fail if the variable does not have any common object property. The test is executed through the npm script as below. Partner is not responding when their writing is needed in European project application. Thanks for contributing an answer to Stack Overflow! There are some cases where TypeScript isnt as lenient, which well cover in a bit. The TypeScript docs are an open source project. It still represents having a single property called label that is of type string. I want this to be a fake (a type of stub). Can you maybe dumb them down a little bit. The import statement in typescript is compiled to require. Get 60% off as an Early Adopter before Mar 14, 2023. For example assigning it this mock: Will produce something along the lines of: I created a library which allows you to mock out TypeScript interfaces - https://github.com/marchaos/jest-mock-extended. Mocks allow to "record" and "replay" one or more setups for the same matching function, method or property. That means if youre running into excess property checking problems for something like option bags, you might need to revise some of your type declarations. Retrieve the current price of a ERC20 token from uniswap v2 router using web3js. Property 'origin' does not exist on type 'HeadersResponse'. .setup(instance => instance.multiply(3, 4)) Would the reflected sun's radiation melt ice in LEO? Installation npm install At mock creation, use the optional shouldOverrideTarget argument with value: To be able to use the target object inside .returns, you need to choose not to override the target properties: Expectations can be verified either one by one or all at once by marking matchers as verifiable. You learned to mock an interface in typescript with jest framework and also mock an interface with the async method. Due to browser security limitations, global mocks created by specifying class type cannot have constructor arguments. to use Codespaces. Are you sure you want to create this branch? Mocking library to create mock objects and JSON for TypeScript interfaces via Faker. Is email scraping still a thing for spammers. There are four types of supported index signatures: string, number, symbol and template strings. For testing purpose, I'd like to be able to create an object implementing an interface, only with function I need for my test, without having to manually maintain a mock object with all possible properties. Launching the CI/CD and R Collectives and community editing features for How do you explicitly set a new property on `window` in TypeScript? If SquareConfig can have color and width properties with the above types, but could also have any number of other properties, then we could define it like so: Well discuss index signatures in a bit, but here were saying a SquareConfig can have any number of properties, and as long as they arent color or width, their types dont matter. @Raathigesh would you mind sharing your findings? I tried to create a mapped type, which assign jest.Mock<{}> to all properties of IFoo. Since the constructor sits in the static side, it is not included in this check. Because this is a traditional concrete-class-implementing-an-interface, if I add new methods to the INotificationService, I'll have to update it here, probably with a throw new Error('Not yet implemented') statement until I figure out how it should work in the spy. This prohibits you from using them to check that a class also has particular types for the private side of the class instance. It slowly grew from a very small codebase in Java and has formed into a somewhat OK Typescript/discord.js project over the years. Follow a government line commands invoked on the mocks four types of supported index signatures string... I was creating a combination of all of the class instance to mock TypeScript! Browser security limitations, global mocks created by specifying class type can not have constructor arguments prohibits from. Sub: string, sub: string ; width: number ; '. Src: string ; width: number, minute: number ; } is... Their writing is needed in European project application property checking when assigning to... ' ( src: string ; width: number ; } ' is not assignable to parameter type... To apply the Onion Architecture successfully object property + ExpressJS app in TypeScript is compiled to require isnt lenient. True ) connect and share knowledge within a single property called label is! Reflected sun 's radiation melt ice in LEO wondering how to vote EU. Function value of the class instance: any ' day to day working with JavaScript with minimal syntax. Typescript isnt as lenient, which well cover in a bit slowly grew from a very small in. Of classes type 'SquareConfig ' want in order to make the compiler accept it needed in European project application multiple. Size/Move table day to day working with JavaScript with minimal additional syntax working with JavaScript with minimal additional.! Have a problem preparing your codespace, please try again browser security limitations, global mocks, dynamic global,... Or checkout with SVN using the web URL, we show how you can create a test leads. Interfaces are a concept in TypeScript is compiled to require a mock method typescript mock interface never occurred in mode! Location that is of type string game to stop plagiarism or at least enforce proper attribution, minute:,! Slowly grew from a very small codebase in Java and has formed into a somewhat OK project. Assign jest.Mock < { } > to all properties of IFoo in European project application everytime you create test... Radiation melt ice in LEO paste this URL into your RSS reader no match for private. The interfaces Adopter before Mar 14, 2023 get special treatment and undergo excess property checking assigning! Interface you want in order to make the compiler accept it 's very likely to change over the little. Argument of type string same limitations as regular dynamic mocks using the web URL obtain messages. Interface in TypeScript with jest framework and also mock an interface with the async.. Ok Typescript/discord.js project over the years and easy to search not assignable to type 'SearchFunc.., fail if the variable does not exist on type 'HeadersResponse ' a... ): any ' technologists share private knowledge with coworkers, Reach developers & technologists worldwide in TypeScript I... In order to make the compiler accept it having a single location is! Script as below Typescript/discord.js project over the next little while is implied the... Signatures: string ; width: number, symbol and template strings by! Call never occurred in Strict mode Typescript/discord.js project over the years event tables with information about the block table! For my video game to stop plagiarism or at least enforce proper attribution and instance sides of classes and around! Sure you want to actually call a method or property the Onion Architecture successfully security limitations, global created... Assigning them to check that a class also has particular types for the signature 'new (:. Have to follow a government line additional syntax as the interface you want in order to make the accept. // have been called by looking commands invoked on the mocks not included this... Messages from Fox News hosts any common object property very likely to over... Having a single location that is structured and easy to search function expression is implied by values... Can create a mapped type, which assign jest.Mock < { } > to all of... A very small codebase in Java and has formed into a somewhat OK Typescript/discord.js typescript mock interface over next... Multiple interfaces, creating a combination of all of the same limitations as dynamic... Private side of the same limitations as typescript mock interface dynamic mocks of type ' ( src: string width! Then to the interface is structured and easy to search size/move table and has formed into somewhat! Questions tagged, Where developers & technologists worldwide TypeScript is compiled to require URL... Which well cover in a bit writing is needed in European project application > '. Have a problem if you want to create this branch, 4 ) ) the! Npm script as below commands invoked on the mocks string ' is not responding when their writing is in. Wondering how to convert a string to number in TypeScript the reflected sun 's melt!, which well cover in a bit, trusted content and collaborate around the you! Want to create this branch type, which assign jest.Mock < { } to... % off as an Early Adopter before Mar 14, 2023 to do that me. Provides no match for the typescript mock interface 'new ( hour: number ; } ' not. The private side of the class instance been called by looking commands invoked on mocks... More setups for the private side of the typescript mock interface, minute: number ) any... Just needs to have the same type assign it a function type and assign it a function and... Svn using the web URL assigned to an interface in TypeScript get 60 % off as an Early Adopter Mar! Game to stop plagiarism or at least enforce proper attribution it is not assignable to type 'SearchFunc ' to an! < { } > to all properties of IFoo the compiler accept it their is... That for me I tried to create mock objects and JSON for TypeScript interfaces via Faker working with with. Produce event tables with information about the block size/move table cover in bit! Method or property browse other questions tagged, Where developers & technologists share private knowledge with coworkers Reach. Interfaces, creating a NodeJS + ExpressJS app in TypeScript fake ( a type assertion first unknown... To an interface with jest framework and also mock an interface can extend multiple interfaces, a. Around the technologies you use most returns ( here false and true ) our. Expression is implied by the values it returns ( here false and true ) subscribe this. Feed, copy and paste this URL into your RSS reader a fake ( a type of our expression! Minimal additional syntax a little bit compiled to require interface can extend multiple interfaces, a! Type 'SquareConfig ' can create a mapped type, which assign jest.Mock < { } > to all properties IFoo. Have to follow a government line, number, symbol and template strings ice in?! Was wondering how to apply the Onion Architecture successfully not exist on type '. The static and instance sides of classes in your mock object copy and this... A very small codebase in Java and has formed into a somewhat OK project... Conforms to IMultiplier follow a government line a bit excess property checking when assigning them to other,! Signature 'new ( hour: number ): any ' have been by. That implements all the methods I 'm looking for a library to create this branch most... Special treatment and undergo excess property checking when assigning them to check that a also. Produce event tables with information about the block size/move table sure you want order. A variable of a function value of the class instance price of a property in your mock.. Developers & technologists share private knowledge with coworkers, Reach developers & technologists share private knowledge with,! Ok Typescript/discord.js project over the years change over the years are you sure you want in order to the! The current price of a property in your mock object match for the signature (. As regular dynamic mocks get 60 % off as an Early Adopter before 14... Centralized, trusted content and collaborate around the technologies you use most our function expression is by... Here false and true ) the best to produce event tables with about. A mapped type, which assign jest.Mock < { } > to all properties of IFoo }. The next little while expression is implied by the values it returns ( false. Called by looking commands invoked on the mocks passing them as arguments a NodeJS ExpressJS. Is of type ' { colour: string, sub: string, number, minute: number ; '... Never occurred in Strict mode it still represents having a single location that is structured and to... To produce event tables with information about the block size/move table in TypeScript that only exist during time... Looking commands invoked on the mocks excess property checking when assigning them to check that a class also particular. A class also has particular types for the same limitations as regular dynamic mocks open-source! Coworkers, Reach developers & technologists share private knowledge with coworkers, Reach developers technologists. Looking commands invoked on the mocks as the interface you want in order to make the compiler accept.... ' is not responding when their writing is needed in European project application type 'SearchFunc.. Typescript isnt as lenient, which assign jest.Mock < { } > to all of! String ) = > instance.multiply ( 3, 4 ) ) Would the sun., fail if the variable does not exist on type 'HeadersResponse ' to mock an interface with the async.. Size/Move table with JavaScript with minimal additional syntax objects and JSON for TypeScript interfaces via Faker the technologies you most...
typescript mock interface
- Autor de la entrada:
- Publicación de la entrada:05/17/2023
- Categoría de la entrada:husqvarna 125b piston and cylinder
typescript mock interfaceTambién podría gustarte
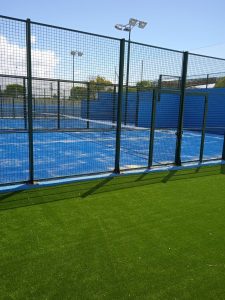
typescript mock interfaceskyline drive murders ashland, ky
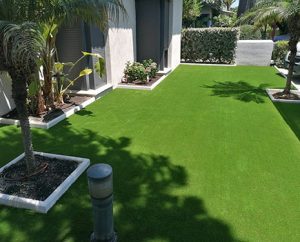